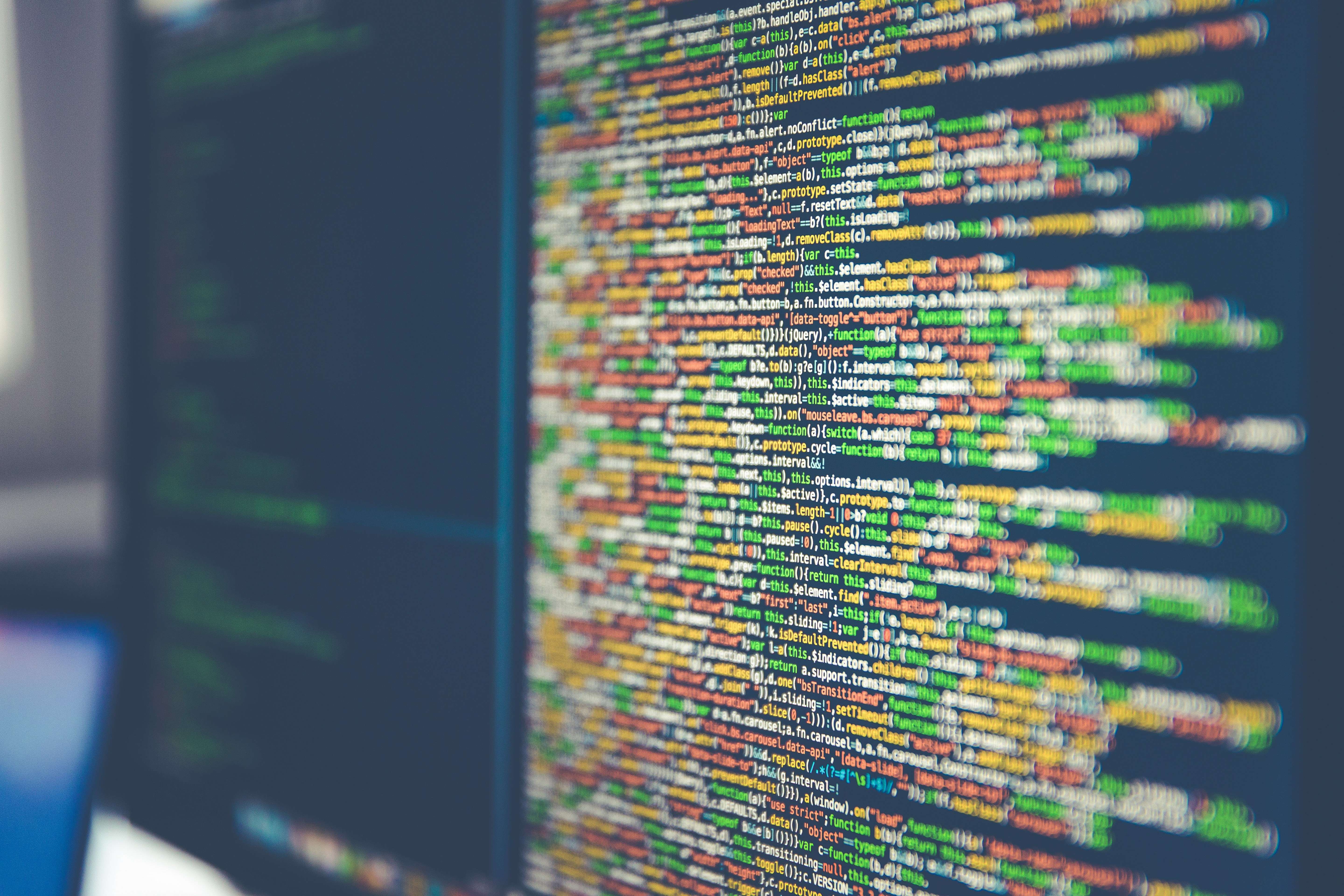
Testing is a fundamental aspect of software development, particularly for web applications where functionality, performance, and security are paramount. Laravel, as a powerful PHP framework, offers a robust testing ecosystem that enables developers to ensure high-quality applications through various testing methodologies. This article will delve into advanced Laravel testing techniques, covering unit testing, feature testing, browser testing, and more, while highlighting best practices for maximizing the effectiveness of your tests.
1. The Importance of Testing in Laravel
Testing plays a crucial role in ensuring that your Laravel application performs as intended. By implementing a comprehensive testing strategy, developers can identify bugs, validate functionality, and ensure that changes do not introduce new issues. Key benefits of testing in Laravel include:
- Confidence in Code Changes: With a well-defined testing suite, developers can make changes and refactor code without fear of breaking existing functionality.
- Reduced Debugging Time: Automated tests help catch errors early in the development process, reducing the time spent debugging issues in production.
- Improved Code Quality: Writing tests encourages developers to follow best practices, leading to cleaner and more maintainable code.
2. Unit Testing in Laravel
Unit testing focuses on testing individual components or functions in isolation, ensuring that they work as intended. Laravel provides a simple and intuitive API for writing unit tests using PHPUnit, making it easy to get started.
Key Features of Laravel’s Unit Testing Framework:
- Assertions: Laravel’s testing framework includes a wide range of assertion methods, enabling developers to verify expected outcomes efficiently. Common assertions include checking for equality, truthiness, and exceptions.
- Mocking: Laravel’s built-in mocking capabilities allow developers to create mock objects, which can simulate the behavior of complex dependencies. This is particularly useful for testing components that rely on external services or databases.
- Database Transactions: Laravel supports database transactions during testing, allowing developers to roll back changes made during a test. This ensures that tests do not affect the database state, providing a clean slate for each test case.
Best Practices for Unit Testing:
- Keep Tests Focused: Each unit test should test a single functionality or behavior. This makes it easier to identify the source of errors when tests fail.
- Use Meaningful Names: Test methods should have descriptive names that indicate what they are testing. This enhances readability and helps other developers understand the purpose of the tests.
- Run Tests Frequently: Integrate unit tests into your development workflow, running them frequently to catch issues early.
3. Feature Testing in Laravel
While unit testing focuses on individual components, feature testing examines the interaction between multiple components or the overall behavior of the application. Laravel makes it easy to write feature tests that simulate user interactions and validate application behavior.
Key Features of Laravel’s Feature Testing:
- HTTP Testing: Laravel’s feature testing capabilities allow developers to simulate HTTP requests and assert the responses. This is essential for testing routes, controllers, and middleware.
- Database Assertions: Developers can easily check the database state after performing actions in feature tests, ensuring that data is being created, updated, or deleted as expected.
- Testing Authentication: Laravel provides built-in support for testing authentication scenarios, allowing developers to validate user login, registration, and permission checks.
Best Practices for Feature Testing:
- Test Real User Scenarios: Focus on simulating real user interactions to ensure that the application behaves as expected from the user’s perspective.
- Keep Tests Organized: Organize feature tests logically, grouping related tests together for better maintainability and readability.
- Utilize Test Data Factories: Laravel’s model factories allow developers to create test data quickly, enabling more comprehensive testing without manual setup.
4. Browser Testing with Laravel Dusk
For applications that require complex user interactions, browser testing is essential. Laravel Dusk provides an elegant solution for automated browser testing, allowing developers to simulate user behavior in a real browser environment.
Key Features of Laravel Dusk:
- Browser Automation: Dusk leverages the ChromeDriver to automate browser interactions, enabling developers to write tests that simulate real user behavior, such as clicking buttons, filling out forms, and navigating between pages.
- Element Assertions: Dusk provides a rich set of assertions to verify the presence, visibility, and content of elements within the browser, ensuring that the user interface functions as intended.
- Screenshot and Console Logging: Dusk allows developers to take screenshots during tests and log console output, making it easier to diagnose issues when tests fail.
Best Practices for Browser Testing:
- Limit Scope: While browser tests provide valuable insights, they can be slower to execute. Limit the number of browser tests to cover critical user interactions while relying on unit and feature tests for broader coverage.
- Run Tests in CI/CD Pipelines: Integrate Dusk tests into your continuous integration and deployment (CI/CD) pipelines to ensure that browser functionality is validated with each deployment.
- Use Headless Mode: For faster test execution, consider running Dusk in headless mode, which allows tests to execute without launching a visible browser window.
5. Performance Testing with Laravel
In addition to functional testing, performance testing is crucial for ensuring that your application can handle expected traffic and provide a smooth user experience. Laravel provides tools for performance testing to identify bottlenecks and optimize application performance.
Key Features of Performance Testing in Laravel:
- Benchmarking: Use Laravel’s testing capabilities to measure the execution time of specific functions or requests, helping identify performance bottlenecks.
- Load Testing: Simulate multiple concurrent users to evaluate how the application performs under load. Tools like Apache JMeter or Laravel's built-in HTTP testing capabilities can be integrated for this purpose.
Best Practices for Performance Testing:
- Monitor Application Metrics: Keep track of key performance metrics such as response time, memory usage, and database query performance during tests to identify areas for optimization.
- Perform Regular Load Testing: Conduct load tests regularly, especially before major releases or when significant changes are made to the application.
6. Continuous Integration and Automated Testing
Integrating automated testing into your development workflow is essential for maintaining high code quality. Continuous integration (CI) tools such as Travis CI, GitHub Actions, or CircleCI can be configured to run your test suite automatically whenever changes are pushed to the repository.
Benefits of Automated Testing in CI:
- Immediate Feedback: Automated tests provide immediate feedback on code changes, allowing developers to catch issues early and address them before they become larger problems.
- Consistent Quality Assurance: By running tests consistently across all code changes, you ensure that your application maintains a high standard of quality throughout its development lifecycle.
Best Practices for CI and Automated Testing:
- Run Tests on Each Pull Request: Configure your CI pipeline to run tests for every pull request, ensuring that only code that passes the test suite is merged into the main branch.
- Monitor Test Coverage: Use tools like PHPUnit’s code coverage reports to monitor test coverage and identify areas of the codebase that require additional testing.
Conclusion
Advanced Laravel testing is essential for building high-quality web applications that perform reliably under various conditions. By leveraging the framework’s powerful testing capabilities, including unit testing, feature testing, browser testing, and performance testing, developers can ensure that their applications meet the highest standards of functionality and user experience.
Implementing best practices in testing, along with continuous integration and automated testing, will empower your development team to deliver robust, maintainable, and scalable applications that meet the needs of users and stakeholders alike. As Laravel continues to evolve, staying abreast of the latest testing techniques and tools will be crucial for maintaining a competitive edge in the ever-changing landscape of web development.