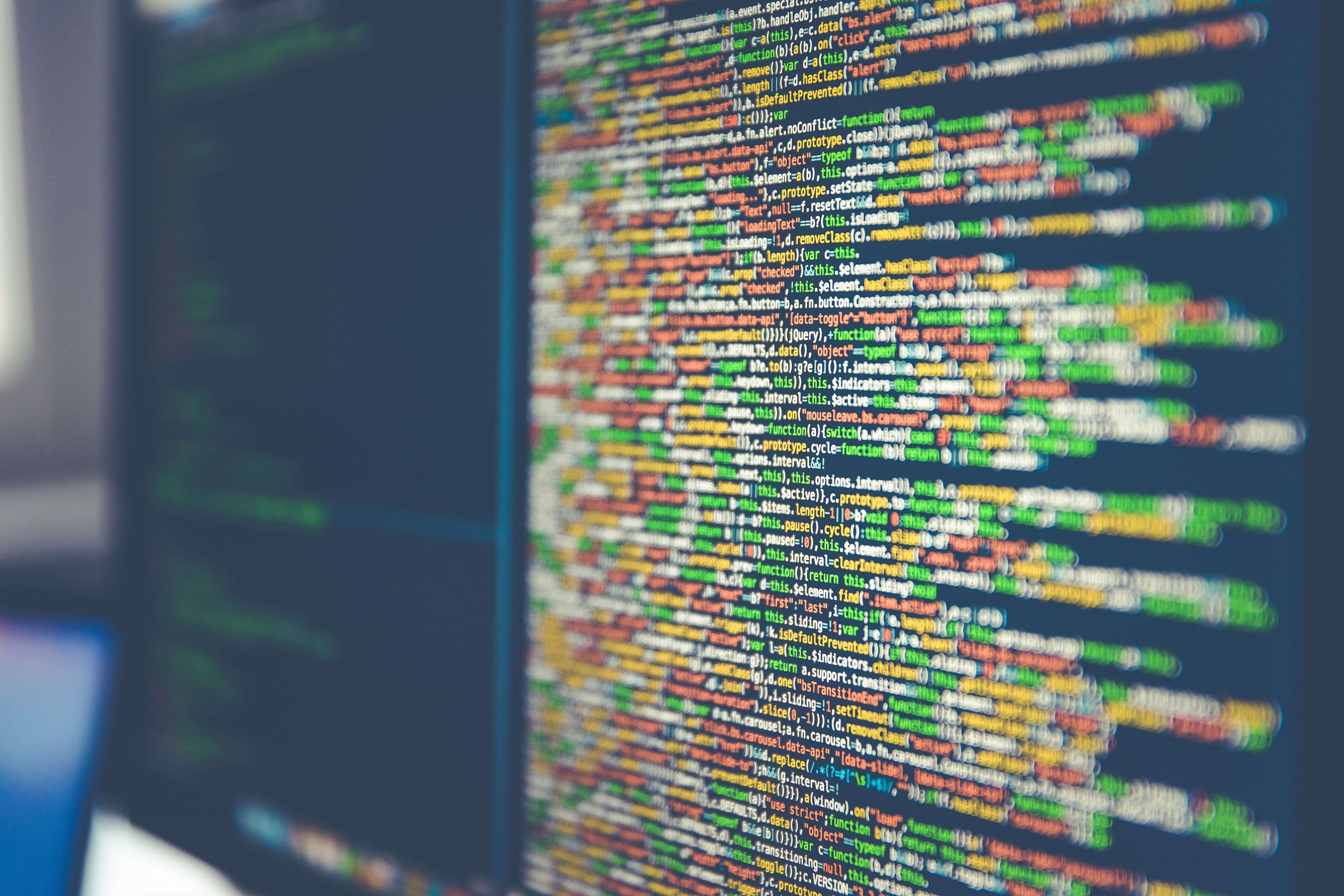
As your web application grows, ensuring scalability becomes essential to maintain performance and handle increasing traffic and user demands. Laravel, one of the most popular PHP frameworks, offers a robust platform for building scalable applications. However, to fully harness its capabilities, developers must follow certain best practices to optimize performance, database management, and the use of Laravel's built-in tools. In this article, we’ll explore the best practices for scaling web applications with Laravel.
1. Efficient Database Queries
Efficient database interaction is crucial for scalability. Poorly optimized queries can slow down your application, especially under heavy traffic. Laravel’s Eloquent ORM simplifies database management, but developers must be mindful of query efficiency.
-
Lazy Loading vs. Eager Loading: Avoid eager loading unless necessary. Lazy loading loads data on-demand, preventing unnecessary data retrieval.
-
Query Caching: Laravel’s built-in cache system allows you to cache query results, reducing database load by serving frequently accessed data from the cache.
-
Pagination: Instead of fetching large datasets in a single query, use pagination to limit data retrieval, especially on pages like product listings or user profiles.
2. Leveraging Laravel Queues
For time-consuming tasks like sending emails, processing files, or handling complex calculations, Laravel’s queue system helps distribute the load by offloading tasks to the background.
-
Background Jobs: Queues allow your application to handle tasks asynchronously, keeping it responsive even under high traffic.
-
Optimized Queue Drivers: Use queue drivers like Redis or Amazon SQS, which are designed to manage large volumes of background jobs efficiently.
-
Job Prioritization: Laravel lets you set priorities for jobs, ensuring critical tasks are handled first while less important ones are deferred.
3. Horizontal Scaling with Laravel Octane
Laravel Octane is a game-changer for scaling. It optimizes performance by running applications in long-lived processes, avoiding the repeated bootstrapping of the framework for each request.
-
Persistent Application Memory: Octane keeps the application in memory, reducing latency and improving response times for high-traffic applications.
-
Server Optimization: Pair Octane with servers like Swoole or RoadRunner to handle thousands of concurrent requests, making your application ideal for real-time systems or apps requiring horizontal scaling across multiple servers.
4. Database Optimization and Sharding
As data grows, optimizing database management becomes crucial. One approach is database sharding, where large datasets are split across multiple databases or servers to balance the load.
-
Sharding and Read/Write Separation: Laravel supports routing queries to different servers, distributing the load to enhance performance.
-
Indexing: Optimize queries by indexing columns frequently used in WHERE clauses or joins. Laravel’s migration system simplifies managing database indexes.
5. Caching Strategies
Caching is one of the most powerful techniques for improving scalability. Laravel supports various caching drivers like Redis, Memcached, and file-based caching.
-
Query and View Caching: Use caching to store frequently accessed data and rendered views, reducing server load by eliminating repetitive database queries and view generation.
-
Cache Tagging: Laravel allows cache grouping through cache tags, making it easy to invalidate specific pieces of cached data when needed.
6. Load Balancing and Autoscaling
When traffic spikes, load balancing helps distribute traffic across multiple servers, ensuring no single server is overwhelmed.
-
Horizontal Scaling: Utilize cloud services like AWS Elastic Load Balancing or Google Cloud Load Balancer to route traffic efficiently during peak times.
-
Autoscaling: Tools like AWS Autoscaling and Google Cloud Autoscaler dynamically add or remove server instances based on real-time traffic, ensuring consistent application availability.
7. Session Management
Efficient session management is key to scalability. Laravel supports storing sessions in Redis or Memcached, which are faster and more reliable than database storage for high-traffic applications.
- Session Clustering: For distributed environments, use session clustering to ensure sessions are accessible across all servers, maintaining a seamless user experience during load balancing.
8. Monitoring and Optimization
Performance monitoring becomes critical as your application scales. Laravel provides tools like Laravel Telescope and Laravel Horizon for tracking application performance, database queries, and job queues.
- APM Tools: Integrate New Relic, Datadog, or Sentry for real-time application performance monitoring, helping you identify slow queries, memory leaks, and other performance bottlenecks before they impact users.
Conclusion: Laravel Makes Scalability Achievable
Laravel’s flexibility and comprehensive ecosystem make it an excellent choice for building scalable web applications. By following best practices—optimizing database queries, leveraging queues, caching data, and monitoring performance—developers can ensure that their applications scale seamlessly to handle growing user demands.
Whether you're building a small app or an enterprise-level platform, Laravel’s scalability features, combined with the right approach, will empower your web application to deliver fast, reliable performance under any load.