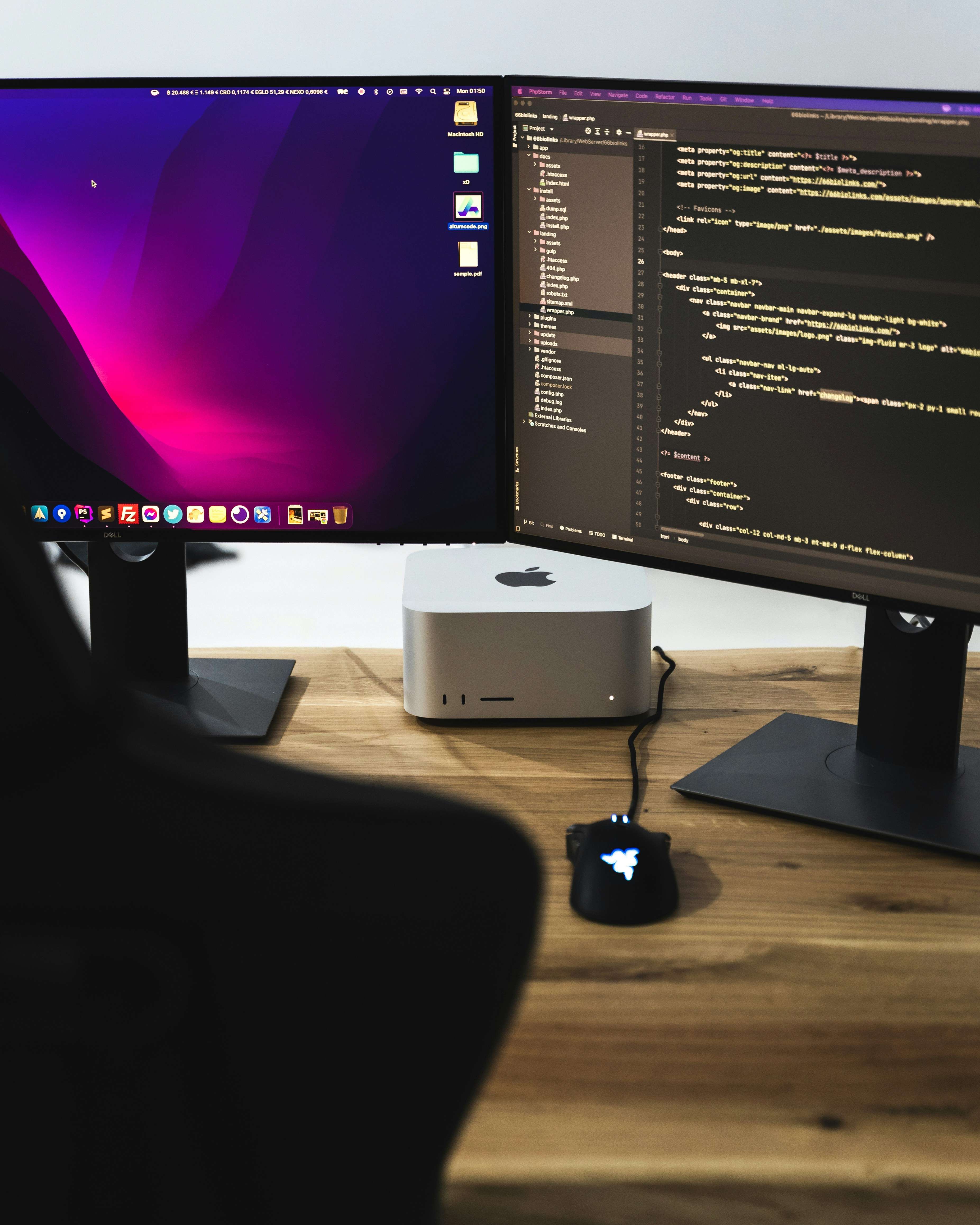
Microservices architecture has emerged as a popular approach for building scalable, flexible applications, especially in complex enterprise environments. This architectural style involves breaking down a large, monolithic application into smaller, loosely coupled services, allowing for independent development, deployment, and scaling. Laravel, with its flexibility and rich set of features, can be effectively used to create scalable applications based on microservices.
In this article, we’ll explore how Laravel fits into microservices architecture, best practices for building scalable applications, and the benefits of combining Laravel with microservices.
Why Microservices?
Monolithic applications bundle the user interface, business logic, and database interactions into a single codebase. While this is effective for smaller projects, larger, more complex applications face several challenges:
- Slow Deployments: Any change requires redeployment of the entire application.
- Scalability Issues: It is difficult to scale specific components independently.
- Development Bottlenecks: Different teams working on the same codebase can create conflicts and delays.
Microservices solve these problems by splitting applications into smaller, independent services, each responsible for a specific function. For instance, in an e-commerce platform, services for user authentication, product catalog, and payment processing can all operate independently, be developed and deployed separately, and scale without affecting one another. This decoupling enhances flexibility, scalability, and development speed.
How Laravel Fits into Microservices Architecture
Though Laravel is commonly used for monolithic applications, it can also serve as a foundation for microservices-based applications due to its modularity and support for APIs. Each microservice can be a standalone Laravel application with its own database, business logic, and API endpoints, communicating with other services through HTTP, RESTful APIs, or messaging protocols.
Lumen, Laravel’s lightweight framework, is a great choice for microservices that require minimal overhead. Lumen maintains Laravel’s core functionality but strips away resource-heavy features, making it ideal for high-performance microservices and APIs.
Decoupling with Laravel: Key Considerations
-
API-First Approach: Each Laravel microservice should expose a RESTful API, allowing other services to interact with it. Laravel Sanctum can be used for API token authentication, and API Resources simplify the serialization of responses.
-
Service Discovery: Microservices need to locate one another without hardcoded URLs. Tools like Consul or Eureka provide service discovery, allowing dynamic service communication.
-
Event-Driven Architecture: Laravel supports event-driven development, making it suitable for asynchronous microservice communication. Services can emit and listen for events using Laravel’s event broadcasting and queue systems, further decoupling the components.
Scalability Through Microservices and Laravel
One of the key advantages of microservices architecture is scalability. Unlike monolithic systems that require scaling the entire application, microservices allow each service to be scaled independently. For instance, the user authentication service can be scaled separately if it experiences heavy traffic without needing to scale the entire system.
Laravel offers several features that enhance scalability:
-
Queues and Job Processing: Laravel’s built-in queue system allows tasks like email sending or file uploads to be deferred, avoiding performance bottlenecks. This is crucial in microservices, where each service can be scaled based on its workload.
-
Caching: Laravel’s support for caching systems like Redis, Memcached, and DynamoDB ensures that frequently accessed data is stored efficiently, reducing load on services. This is particularly important in microservices, where multiple services might rely on shared data.
-
Load Balancing and Autoscaling: Laravel microservices can be deployed on cloud platforms such as AWS, Google Cloud, or Azure, which provide load balancing and autoscaling features. This ensures that services dynamically adjust to traffic demands, optimizing performance.
Database Management in Microservices
In a monolithic application, a single database serves the entire system, leading to tight coupling. Microservices, however, follow the database-per-service pattern, ensuring complete independence between services. Each Laravel microservice can have its own database, managed through Eloquent ORM with independent models, migrations, and connections.
When services need to share data, APIs can facilitate the communication. More advanced patterns like Event Sourcing and CQRS (Command Query Responsibility Segregation) can be used to maintain data consistency without tight coupling.
Using Laravel Octane for High-Performance Microservices
Laravel Octane optimizes Laravel for high-performance environments by using long-lived processes with Swoole or RoadRunner. These tools keep the application in memory, reducing the time and resources spent on bootstrapping Laravel with each request. This is particularly beneficial for high-traffic microservices, such as real-time applications or those handling a high volume of API requests.
Service-Oriented Communication: API Gateways and Message Queues
Communication between microservices is crucial, and Laravel can be used to implement an API Gateway, a centralized entry point for external requests. API gateways route requests to the appropriate services, handling rate-limiting, caching, and authentication.
For asynchronous communication, Laravel supports event broadcasting and message queues such as RabbitMQ or Kafka. This decouples services further, allowing them to process messages at their own pace without direct API calls.
Monitoring and Observability in Laravel Microservices
As the number of microservices grows, it’s important to have robust monitoring. Tools like Prometheus, Grafana, and AWS CloudWatch can be integrated with Laravel to monitor health, performance, and errors. Centralized logging with services like Logstash or the ELK stack ensures logs from multiple services can be analyzed efficiently, providing insights into system performance and identifying issues early.
Conclusion: Combining Laravel and Microservices for Scalable Applications
The combination of Laravel and microservices offers a powerful approach to building scalable, maintainable applications. Microservices improve flexibility and performance by decoupling components, while Laravel’s rich feature set—queues, caching, API support, and more—makes it a great fit for microservices-based systems.
As demand for scalable, cloud-native applications grows, leveraging Laravel and microservices ensures your applications are well-equipped to handle the challenges of modern web development. Whether you’re building enterprise applications or real-time services, this combination provides the scalability and performance needed for success.