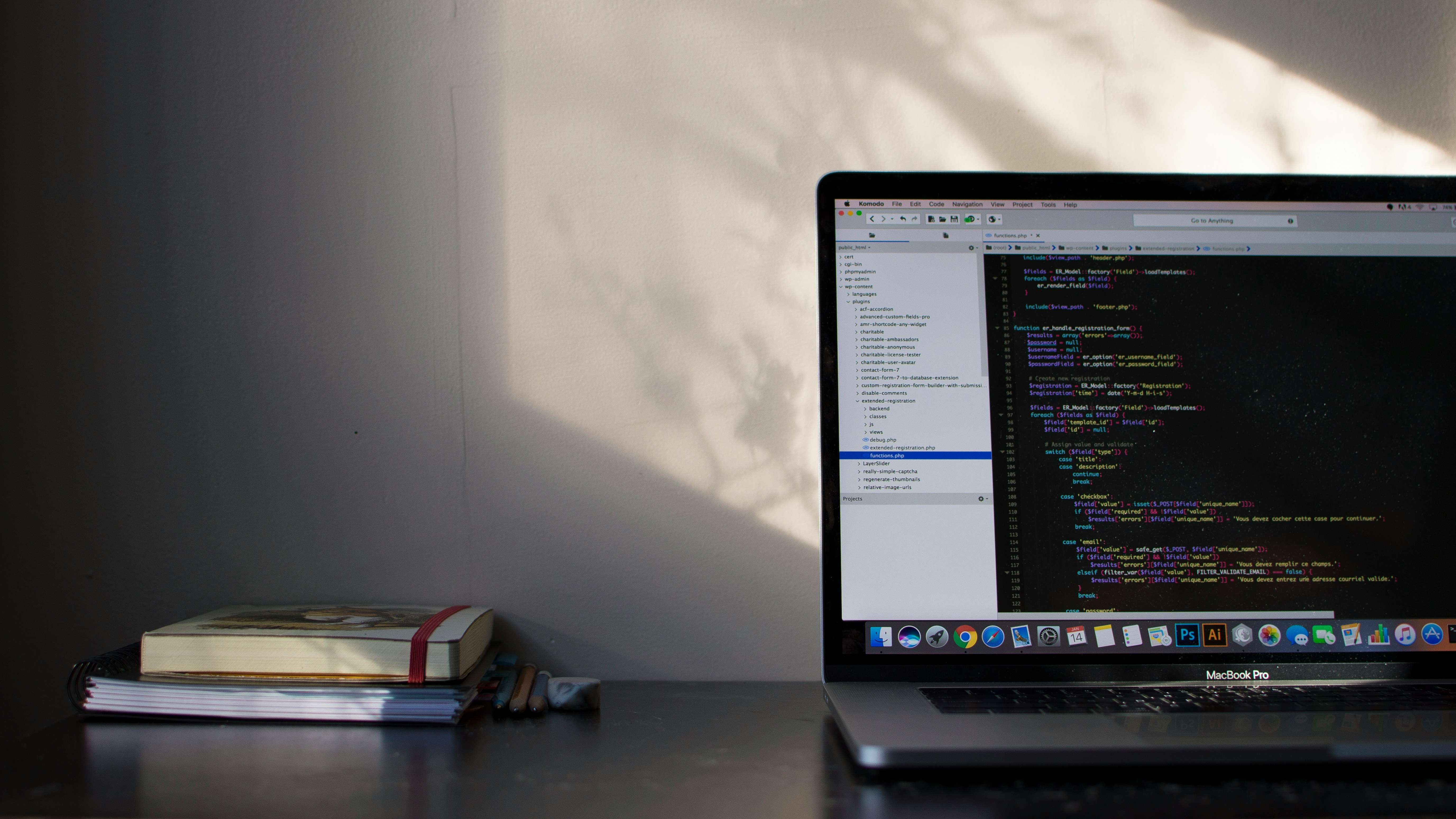
Laravel is renowned for its elegant syntax and rich features, making it a favorite among developers for building modern web applications. However, as applications grow in complexity and user traffic increases, performance optimization becomes crucial. This article explores essential tips and techniques for optimizing the performance of Laravel applications, ensuring they run smoothly and efficiently.
1. Leverage Caching
One of the most effective ways to enhance performance is through caching. Laravel supports various caching mechanisms, allowing you to store data temporarily to reduce the load on your database.
Types of Caching in Laravel:
- Route Caching: Laravel can cache your routes to speed up the route registration process.
- Configuration Caching: Caching your configuration files will reduce the time taken to load the configuration for your application.
- View Caching: Compiling views can be time-consuming, but Laravel caches compiled views automatically, and you can pre-compile them as needed.
- Data Caching: Utilize Laravel’s built-in caching to cache query results or complex calculations, minimizing repetitive database calls.
2. Optimize Your Database Queries
Efficient database queries are vital for performance. Laravel provides several tools to optimize database interactions.
Techniques for Database Optimization:
- Eager Loading: This reduces the number of queries executed by loading relationships when the main model is retrieved, minimizing the N+1 query problem.
- Query Optimization: Use Laravel’s query builder to optimize SQL queries by selecting only necessary columns and avoiding complex queries that can be broken down.
- Database Indexing: Ensure your database tables are properly indexed to improve search efficiency and reduce query time, especially for large datasets.
3. Use Queues for Time-Consuming Tasks
Long-running processes can slow down your application. Offloading these tasks to a queue can significantly improve response times.
Implementing Queues in Laravel:
- Job Queues: Laravel provides a powerful job queue system that allows you to queue tasks such as sending emails or processing uploads.
- Queue Workers: Use workers to process queued jobs in the background, allowing your application to respond quickly to user requests.
- Database and Redis: Laravel supports various queue backends, including database and Redis, providing flexibility based on your application’s needs.
4. Utilize Route Middleware Wisely
Middleware can affect application performance, especially if they perform heavy computations or database calls. Optimize your middleware usage to ensure they are lightweight and only applied where necessary.
Tips for Middleware Optimization:
- Limit Middleware: Only apply middleware to routes that need them, reducing overhead and improving response times.
- Combine Middleware: If multiple middleware are doing similar tasks, consider combining them to minimize processing time.
5. Optimize Assets
Optimizing assets like CSS, JavaScript, and images can drastically improve loading times.
Asset Optimization Techniques:
- Minification: Use tools to minify CSS and JavaScript files, reducing file size and improving load times.
- Image Optimization: Use image compression tools to reduce image sizes without losing quality, which helps in faster page loads.
- CDN Usage: Implement a Content Delivery Network (CDN) to serve static assets, caching them at various geographical locations to ensure faster load times for users.
6. Use PHP OPcache
OPcache is a caching engine built into PHP that can greatly enhance performance by storing precompiled script bytecode in memory, reducing script execution times.
Enabling OPcache:
- Ensure OPcache is enabled in your PHP configuration, as it helps improve the speed of your Laravel application.
7. Profile and Monitor Performance
Understanding where bottlenecks occur is essential for optimization. Use profiling tools to monitor and analyze your application’s performance.
Tools for Monitoring Performance:
- Laravel Telescope: A debugging assistant for Laravel that provides insight into requests, exceptions, database queries, and more.
- Laravel Debugbar: This package displays a debug toolbar in your application, showing details about queries, route information, and more.
- Third-Party Services: Consider using services like New Relic or Blackfire to gain in-depth performance insights.
8. Implement HTTP/2 and SSL
Modern web performance best practices recommend using HTTP/2 and enabling SSL. HTTP/2 allows multiple requests to be sent over a single connection, significantly improving loading times.
Steps to Implement:
- Use HTTPS: Ensure your application runs over HTTPS for secure connections.
- Enable HTTP/2: Check with your hosting provider to enable HTTP/2 support, which enhances the performance of your application, especially for resource-heavy pages.
9. Review Your Application’s Architecture
Sometimes, the architecture of your application can lead to performance issues. Regularly review your application design and refactor when necessary.
Considerations for Architectural Improvements:
- Modular Design: Break your application into smaller modules or services to allow for independent scaling and improved performance.
- Service-Oriented Architecture (SOA): If your application grows in complexity, consider adopting a service-oriented approach to improve maintainability and scalability.
10. Stay Updated with Laravel Releases
Laravel continually improves performance with each release. Stay updated with the latest features and improvements by regularly upgrading your Laravel version.
Benefits of Upgrading:
- Performance Enhancements: Each new version typically includes performance optimizations and new features that can benefit your application.
- Security Fixes: Upgrading ensures your application remains secure and compliant with the latest standards.
Conclusion
Optimizing Laravel applications for better performance involves a combination of caching strategies, efficient database interactions, queue management, and regular monitoring. By implementing these tips and techniques, developers can significantly enhance their applications' speed and responsiveness. As web applications continue to evolve, staying informed about best practices and leveraging Laravel's robust features will be essential for creating high-performance applications that delight users and meet business needs.